










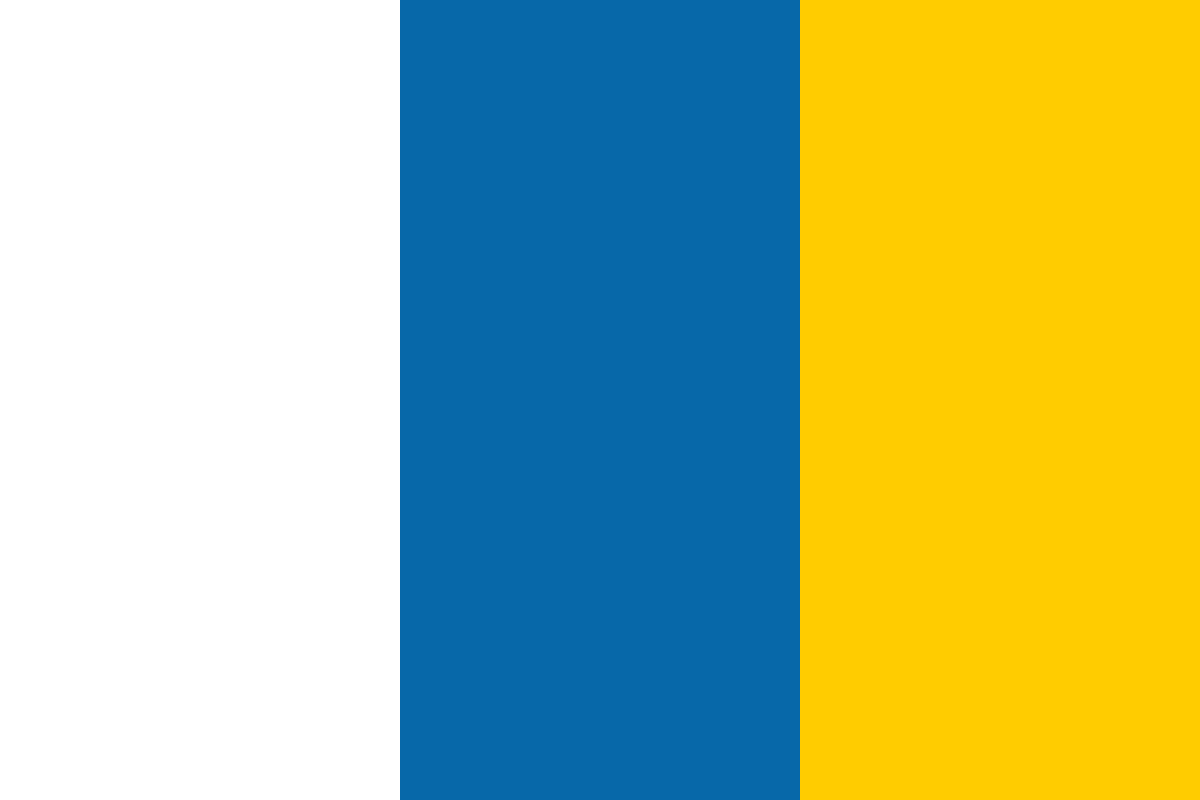

































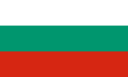








Now that you know the basic primitive data types in JavaScript, it's time to move to a more interesting data structure—the array.
To declare a variable that contains an empty array, you use square brackets with nothing between them:
>>> var a = [];
>>> typeof a;
"object"
typeof returns "object", but don't worry about this for the time being, we'll get to that when we take a closer look at objects.
To define an array that has three elements, you do this:
>>> var a = [1,2,3];
When you simply type the name of the array in the Firebug console, it prints the contents of the array:
>>> a
[1, 2, 3]
So what is an array exactly? It's simply a list of values. Instead of using one variable to store one value, you can use one array variable to store any number of values as elements of the array. Now the question is how to access each of these stored values?
The elements contained in an array are indexed with consecutive numbers starting from zero. The first element has index (or position) 0, the second has index 1 and so on. Here's the three-element array from the previous example:
Index |
Value |
0 |
1 |
1 |
2 |
2 |
3 |
In order to access an array element, you specify the index of that element inside square brackets. So a[0] gives you the first element of the array a, a[1] gives you the second, and so on.
>>> a[0]
1
>>> a[1]
2
Using the index, you can also update elements of the array. The next example updates the third element (index 2) and prints the contents of the new array.
>>> a[2] = 'three';
"three"
>>> a
[1, 2, "three"]
You can add more elements, by addressing an index that didn't exist before.
>>> a[3] = 'four';
"four"
>>> a
[1, 2, "three", "four"]
If you add a new element, but leave a gap in the array, those elements in between are all assigned the undefined value. Check out this example:
>>> var a = [1,2,3];
>>> a[6] = 'new';
"new"
>>> a
[1, 2, 3, undefined, undefined, undefined, "new"]
In order to delete an element, you can use the delete operator. It doesn't actually remove the element, but sets its value to undefined. After the deletion, the length of the array does not change.
>>> var a = [1, 2, 3];
>>> delete a[1];
true
>>> a
[1, undefined, 3]
An array can contain any type of values, including other arrays.
>>> var a = [1, "two", false, null, undefined];
>>> a
[1, "two", false, null, undefined]
>>> a[5] = [1,2,3]
[1, 2, 3]
>>> a
[1, "two", false, null, undefined, [1, 2, 3]]
Let's see an example where you have an array of two elements, each of them being an array.
>>> var a = [[1,2,3],[4,5,6]];
>>> a
[[1, 2, 3], [4, 5, 6]]
The first element of the array is a[0] and it is an array itself.
>>> a[0]
[1, 2, 3]
To access an element in the nested array, you refer to the element index in another set of square brackets.
>>> a[0][0]
1
>>> a[1][2]
6
Note also that you can use the array notation to access individual characters inside a string.
>>> var s = 'one';
>>> s[0]
"o"
>>> s[1]
"n"
>>> s[2]
"e"
There are more ways to have fun with arrays, but let's stop here for now, remembering that: