










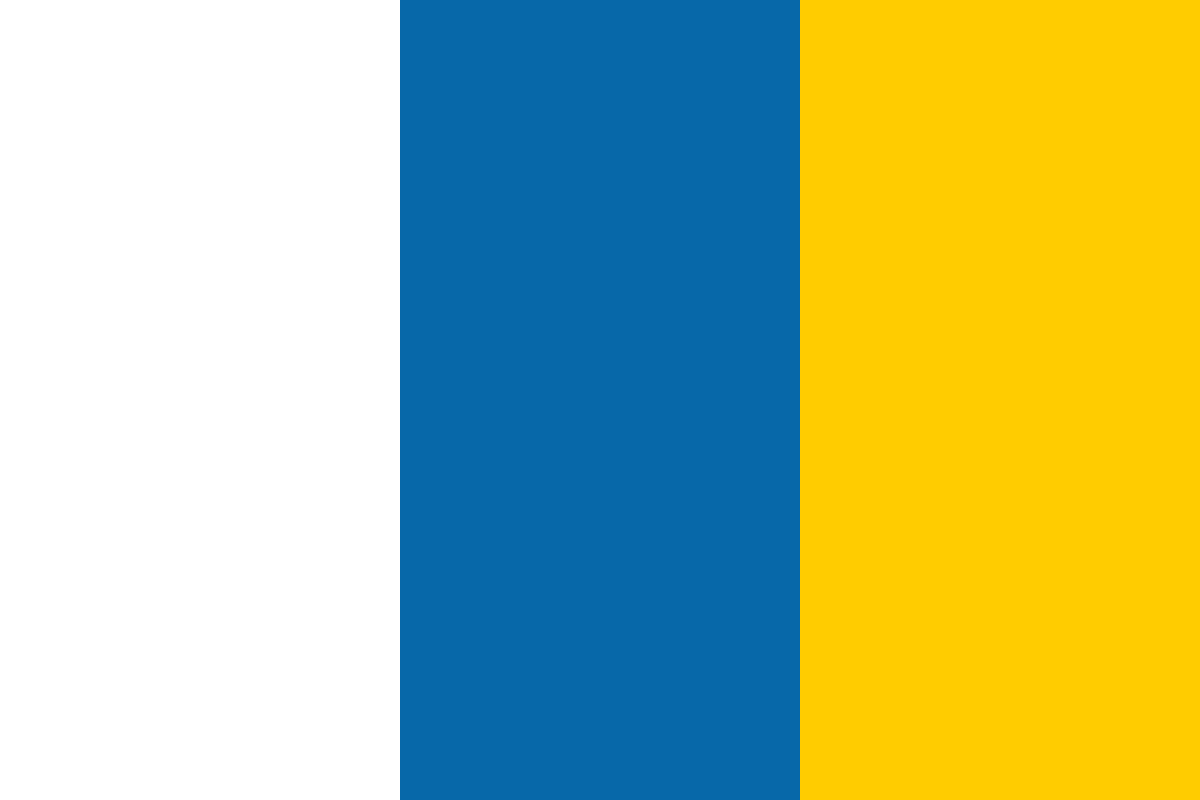

































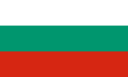








In this article, Marco Schwartz and Stefan Buttigieg, the authors of the Arduino Android Blueprints, we are going to use most of the concepts we have learned to control a mobile robot via an Android app. The robot will have two motors that we can control, and also an ultrasonic sensor in the front so that it can detect obstacles. The robot will also have a BLE chip so that it can receive commands from the Android app.
The following will be the major takeaways:
(For more resources related to this topic, see here.)
We are first going to assemble the robot itself, and then see how to connect the Bluetooth module and the ultrasonic sensor. To give you an idea of what you should end up with, the following is a front-view image of the robot when fully assembled:
The following image shows the back of the robot when fully assembled:
The first step is to assemble the robot chassis. To do so, you can watch the DFRobot assembly guide at https://www.youtube.com/watch?v=tKakeyL_8Fg.
Then, you need to attach the different Arduino boards and shields to the robot. Use the spacers found in the robot chassis kit to mount the Arduino Uno board first. Then put the Arduino motor shield on top of that. At this point, use the screw header terminals to connect the two DC motors to the motor shield. This is how it should look at this point:
Finally, mount the prototyping shield on top of the motor shield.
We are now going to connect the BLE module and the ultrasonic sensor to the Arduino prototyping shield. The following is a schematic diagram showing the connections between the Arduino Uno board (done via the prototyping shield in our case) and the components:
Now perform the following steps:
To review the pin order on the URM37 module, you can check the official DFRobot documentation at http://www.dfrobot.com/wiki/index.php?title=URM37_V3.2_Ultrasonic_Sensor_(SKU:SEN0001).
The following is a close-up image of the prototyping shield with the BLE module connected:
We are now going to write a sketch to test the different functionalities of the robot, first without using Bluetooth. As the sketch is quite long, we will look at the code piece by piece. Before you proceed, make sure that the battery is always plugged into the robot. Now perform the following steps:
#includ e <aREST.h>
int speed_motor1 = 6; int speed_motor2 = 5; int direction_motor1 = 7; int direction_motor2 = 4;
int distance_sensor = A3;
aREST rest = aREST();
int distance;
Serial.begin(115200);
rest.variable("distance",&distance);
rest.function("forward",forward); rest.function("backward",backward); rest.function("left",left); rest.function("right",right); rest.function("stop",stop);
rest.set_id("001"); rest.set_name("mobile_robot");
distance = measure_distance(distance_sensor);
rest.handle(Serial);
void send_motor_command(int speed_pin, int direction_pin, int pwm, boolean dir) { analogWrite(speed_pin, pwm); // Set PWM control, 0 for stop, and 255 for maximum speed digitalWrite(direction_pin, dir); // Dir set the rotation direction of the motor (true or false means forward or reverse) }
int forward(String command) { send_motor_command(speed_motor1,direction_motor1,100,1); send_motor_command(speed_motor2,direction_motor2,100,1); return 1; }
int backward(String command) { send_motor_command(speed_motor1,direction_motor1,100,0); send_motor_command(speed_motor2,direction_motor2,100,0); return 1; }
int left(String command) { send_motor_command(speed_motor1,direction_motor1,75,0); send_motor_command(speed_motor2,direction_motor2,75,1); return 1; }
int stop(String command) { send_motor_command(speed_motor1,direction_motor1,0,1); send_motor_command(speed_motor2,direction_motor2,0,1); return 1; }
There is also a function to make the robot turn right, which is not detailed here.
We are now going to test the robot. Before you do anything, ensure that the battery is always plugged into the robot. This will ensure that the motors are not trying to get power from your computer USB port, which could damage it.
Also place some small support at the bottom of the robot so that the wheels don't touch the ground. This will ensure that you can test all the commands of the robot without the robot moving too far from your computer, as it is still attached via the USB cable.
Now you can upload the sketch to your Arduino Uno board. Open the serial monitor and type the following:
/forward
This should make both the wheels of the robot turn in the same direction. You can also try the other commands to move the robot to make sure they all work properly. Then, test the ultrasonic distance sensor by typing the following:
/distance
You should get back the distance (in centimeters) in front of the sensor:
{"distance": 24, "id": "001", "name": "mobile_robot", "connected": true}
Try changing the distance by putting your hand in front of the sensor and typing the command again.
Now that we have made sure that the robot is working properly, we can write the final sketch that will receive the commands via Bluetooth. As the sketch shares many similarities with the test sketch, we are only going to see what is added compared to the test sketch. We first need to include more libraries:
#include <SPI.h> #include "Adafruit_BLE_UART.h" #include <aREST.h>
We also define which pins the BLE module is connected to:
#define ADAFRUITBLE_REQ 10 #define ADAFRUITBLE_RDY 2 // This should be an interrupt pin, on Uno thats #2 or #3 #define ADAFRUITBLE_RST 9
We have to create an instance of the BLE module:
Adafruit_BLE_UART BTLEserial = Adafruit_BLE_UART(ADAFRUITBLE_REQ, ADAFRUITBLE_RDY, ADAFRUITBLE_RST);
In the setup() function of the sketch, we initialize the BLE chip:
BTLEserial.begin();
In the loop() function, we check the status of the BLE chip and store it in a variable:
BTLEserial.pollACI(); aci_evt_opcode_t status = BTLEserial.getState();
If we detect that a device is connected to the chip, we handle the incoming request with the aREST library, which will allow us to use the same commands as before to control the robot:
if (status == ACI_EVT_CONNECTED) { rest.handle(BTLEserial); }
You can now upload the code to your Arduino board, again by making sure that the battery is connected to the Arduino Uno board via the power jack. You can now move on to the development of the Android application to control the robot.
In this article, we managed to create our very own mobile robot together with a companion Android application that we can use to control our robot.
We achieved this step by step by setting up an Arduino-enabled robot and coding the companion Android application. It uses the BLE software and hardware of an Android physical device running on Android 4.3 or higher.