










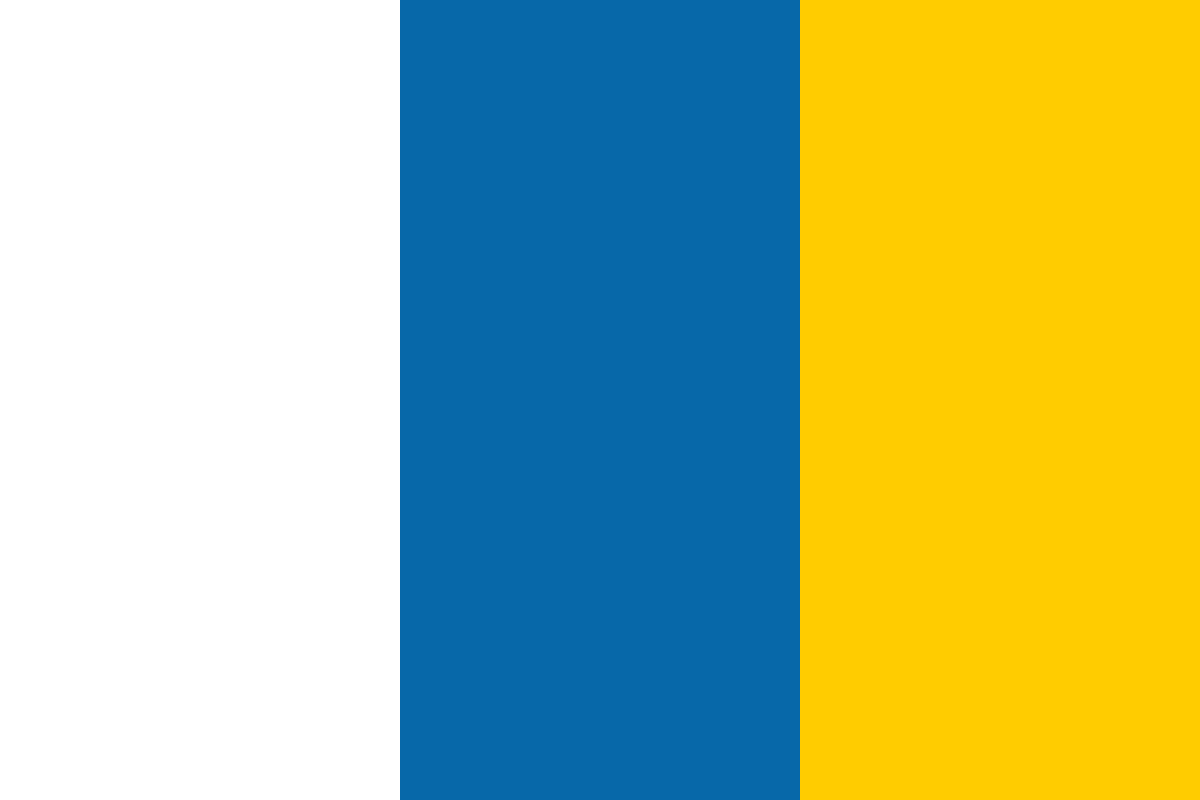

































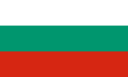








So, you’ve started a web company. You’ve even attracted one or two solid clients. But now you have to produce, and you have to produce fast. If you’ve been in this situation, then we have something in common. This is where I found myself a few months ago. A caveat: I am a self-taught web developer in an absolute sense. Self-taught or not, in August of 2015 I found myself charged with creating a fully functional blogging app for an author.
Needless to say, I was in over my head. I was aware of Node.js, because that had been the backend for the very simple static content site my company had produced first. I was aware of database concepts and I did know a reasonable amount of JavaScript, but I felt ill prepared to pull of these tools together in a cohesive fashion. Luckily for me it was 2015 and not 1998. Today, web developers are blessed with tools that make the development of websites and web apps a breeze. After some research, I decided to use Angular.js to control the frontend behavior of the website, Node.js with Express.js as the backend, and Firebase to hold the data. Let me walk you through the steps I used to get started.
First of all, if you aren’t using Express.js on top of Node.js for your backend in development you should start. Node.js was written in C, C++ and JavaScript by Ryan Dahl in 2009. This multiplatform runtime environment for JavaScript is fast, open-source, and easy to learn, because odds are you already know JavaScript. Using Express.js and the Express-Generator in congress with Node.js makes development quite simple. Express.js is Node middleware. In simple terms, Express.js makes your life a whole lot easier by doing most of the work for you. So, let’s build our backend.
First, install Node.js and NPM on your system. There are a variety of online resources to complete this step. Then, using NPM, install the Express application generator.
$ npm install express-generator -g
Once we have Node.js and the Express generator installed, get to your development folder and execute the following commands to build the skeleton of your web app’s backend:
$ express app-name –e
I use the –e flag to set the middleware to use ejs files instead of the default jade files. I prefer ejs to jade but you might not. This command will produce a subdirectory called app-name in your current directory. If you navigate into this directory and type the commands
$ npm install
$ npm start
and then navigate in a browser to http://localhost:3000 you will see the basic welcome page auto generated by Express. There are thousands of great things about Node.js and Express.js and I will leave them to be discovered by you as you continue to use these tools. Right now, we are going to get Firebase connected to our server. This can serve as general instructions for installing and using Node modules as well.
Head over to firebase.com and create a free account. If you end up using Firebase for a commercial app you will probably want to upgrade to a paid account, but for now the starter account should be fine.
Once you get your Firebase account setup, create a Firebase instance using their online interface. Once this is done get back to your backend code to connect the Firebase to your server.
First install the Firebase Node module.
$ npm install firebase --save
Make sure to use the --save flag because this puts a new line in your packages.json file located in the root of the web server. This means that if you type npm install, as you did earlier, NPM will know that you added firebase to your webserver and install it if it is not already present.
Now open the index.js file in the routes folder in the root of your Node app. At the top of this folder, put in the line
var Firebase = require(‘firebase’);
This pulls the Firebase module you installed into your code. Then to create a connection to the account you just created on Firebase[MA1] ,put in the following line of code:
var FirebaseRef = new Firebase("https://APP-NAME.firebaseio.com/");
Now, to take a snapshot in JSON of your Firebase and store it in an object, include the following lines
var FirebaseContent = {};
FirebaseRef.on("value", function(snapshot) {
FirebaseContent = snapshot.val();
}, function(errorObject) {
console.log("The read failed: " + errorObject.code);
});
FirebaseContent is now a JavaScript object containing your complete Firebase data.
Now let’s get Angular.js hooked up to the frontend of the website and then it’s time for you to start developing. Head over to angularjs.org and download the source code or get the CDN. We will be using the CDN. Open the file index.ejs in the views directory in your Node app’s root. Modify the <head> tag, adding the CDN.
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0-rc.1/angular.min.js"></script>
</head>
This allows you to use the Angular.js tools. Angular.js uses controllers to control your app. Let’s make your angular app and connect a controller.
Create a file called myapp.js in your public/javascripts directory. In myapp.js include the following
angular.module(“myApp”,[]);
This file will grow but for now this is all you need. Now create a file in the same directory called myController.js and put this code into it.
Angular.module(“myApp”).controller(‘myController’,['$scope',function($scope){
$scope.jsVariable = 'Controller is working';
}])
Now modify the index.ejs file again.
<html ng-app=“myApp”>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.0-rc.1/angular.min.js"></script>
<script src="/javascripts/myApp.js"></script>
<script src="/javascripts/myController.js"></script>
</head>
<body ng-controller= “myController”>
<h1> {{jsVariable}} </h1>
</body>
</html>
If you start your app again and go back to http://localhost:3000 you should see that your controller is now controlling the contents of the first heading.
This is just a basic setup and there is much more you will learn along the way. Speaking from experience, taking the time to learn these tools and put them into use will make your development faster and easier and your results will be of much higher quality.
Erik was born and raised in Missoula, Montana. He attended the University of Montana and received a degree in economics. Along the way, he discovered the value of technology in our modern world, especially to businesses. He is currently seeking a master's degree in economics from the University of Montana and his research focuses on the economics of gender empowerment in the developing world.
During his professional life, he has worn many hats – from cashier, to barista, to community organizer. He feels that Montana offers a unique business environment that is often best understood by local businesses. He started his company, Duplovici, with his friends in an effort to meet the unique needs of Montana businesses, non-profits, and individuals. He believes technology is not simply an answer to profit maximization for businesses: by using internet technologies we can unleash human creativity through collective action and the arts, as well as business ventures.
He is also the proud father of two girls and has a special place in his heart for getting girls involved with technology and business.