










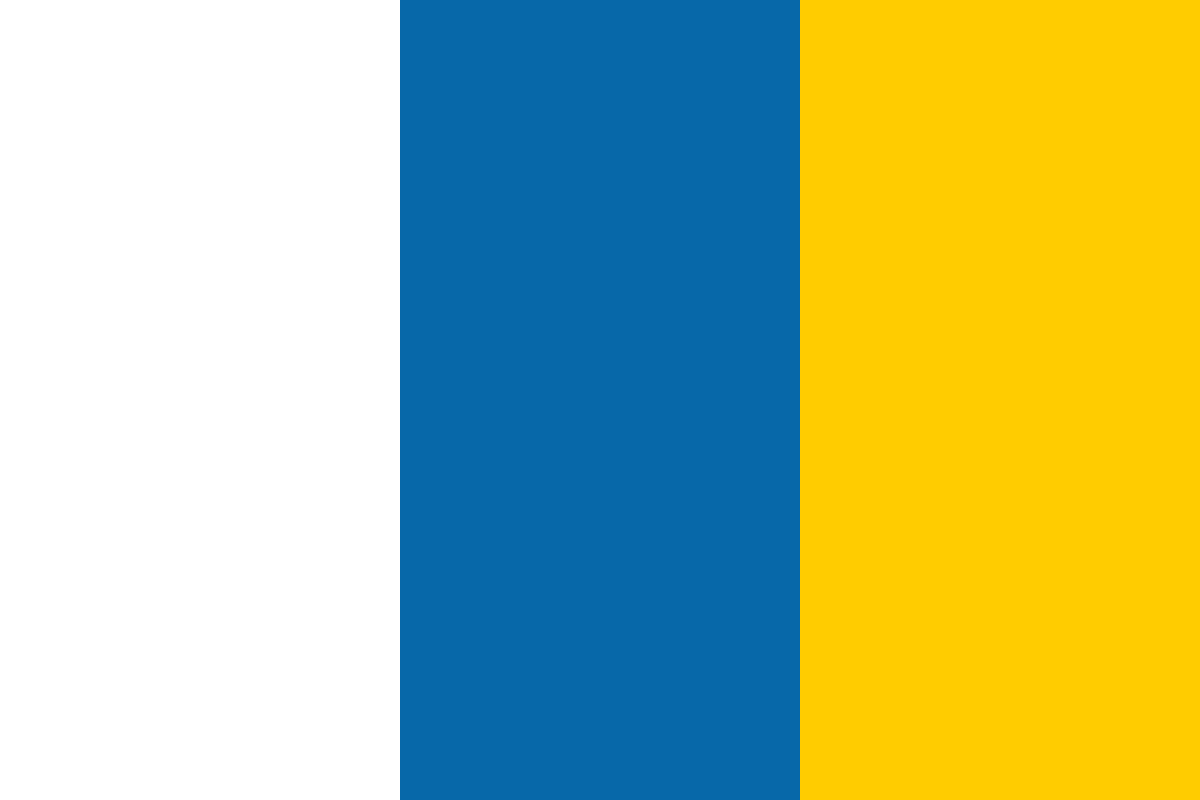

































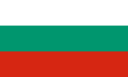








Angular is a mature technology with introduction to new way to build applications. Think of Angular Pipes as modernized version of filters comprising functions or helps used to format the values within the template. Pipes in Angular are basically extension of what filters were in Angular v1. There are many useful built-in Pipes we can use easily in our templates. In today’s tutorial we will learn about Built-in Pipes as well as create our own custom user-defined pipe.
Pipes allows us to format the values within the view of the templates before it's displayed.
For example, in most modern applications, we want to display terms, such as today, tomorrow, and so on and not system date formats such as April 13 2017 08:00. Let's look more real-world scenarios.
You want the hint text in the application to always be lowercase? No problem; define and use lowercasePipe. In weather app, if you want to show month name as MAR or APR instead of full month name, use DatePipe.
Cool, right? You get the point. Pipes helps you add your business rules, so you can transform the data before it's actually displayed in the templates.
A good way to relate Angular Pipes is similar to Angular 1.x filters. Pipes do a lot more than just filtering.
We have used Angular Router to define Route Path, so we have all the Pipes functionalities in one page. You can create in same or different apps. Feel free to use your creativity.
In Angular 1.x, we had filters--Pipes are replacement of filters.
The pipe operator is defined with a pipe symbol (|) followed by the name of the pipe:
{{ appvalue | pipename }}
The following is an example of a simple lowercase pipe:
{{"Sridhar Rao" | lowercase}}
In the preceding code, we are transforming the text to lowercase using the lowercase pipe. Now, let's write an example component using the lowercase pipe example:
@Component({
selector: 'demo-pipe', template: `
Author name is {{authorName | lowercase}}
`
})
export class DemoPipeComponent {
authorName = 'Sridhar Rao';
}
Let's analyze the preceding code in detail:
Run the preceding code, and you should see the screenshot shown as follows as an output:
Well done! In the preceding example, we have used a Built-in Pipe. In next sections, you will learn more about the Built-in Pipes and also create a few custom Pipes.
Note that the pipe operator only works in your templates and not inside controllers.
Angular Pipes are modernized version of Angular 1.x filters. Angular comes with a lot of predefined Built-in Pipes.
We can use them directly in our views and transform the data on the fly.
The following is the list of all the Pipes that Angular has built-in support for: DatePipe
In the following sections, let's implement and learn more about the various pipes and see them in action.
DatePipe, as the name itself suggest, allows us to format or transform the values that are date related.
DatePipe can also be used to transform values in different formats based on parameters passed at runtime.
The general syntax is shown in the following code snippet:
{{today | date}} // prints today's date and time
{{ today | date:'MM-dd-yyyy' }} //prints only Month days and year
{{ today | date:'medium' }}
{{ today | date:'shortTime' }} // prints short format
Let's analyze the preceding code snippets in detail:
Now, let's create a complete example of the date pipe component. The following is the code snippet for implementing the DatePipe component:
import { Component } from '@angular/core';
@Component({
template: `
<h5>Built-In DatePipe</h5>
<ol>
<li>
<strong>DatePipe example expression</strong>
<p>Today is {{today | date}}
<p>{{ today | date:'MM-dd-yyyy' }}
<p>{{ today | date:'medium' }}
<p>{{ today | date:'shortTime' }}
</li>
</ol>
`,
})
Let's analyze the preceding code snippet in detail:
Run the application, and we should see the output as shown in the following screenshot:
We learned the date pipe in this section. In the following sections, we will continue to learn and implement other Built-in Pipes and also create some custom user-defined pipes.
In this section, you will learn about yet another Built-in Pipe--DecimalPipe.
DecimalPipe allows us to format a number according to locale rules. DecimalPipe can also be used to transform a number in different formats.
The general syntax is shown as follows:
appExpression | number [:digitInfo]
In the preceding code snippet, we use the number pipe, and optionally, we can pass the parameters.
Let's look at how to create a DatePipe implementing decimal points. The following is an example code of the same:
import { Component } from '@angular/core';
@Component({
template: `
state_tax (.5-5): {{state_tax | number:'.5-5'}}
state_tax (2.10-10): {{state_tax | number:'2.3-3'}}
`,
})
export class PipeComponent {
state_tax: number = 5.1445;
}
Let's analyze the preceding code snippet in detail:
The output of the preceding pipe component example is given as follows:
Undoubtedly, number pipe is one of the most useful and used pipe across various applications. We can transform the number values specially dealing with decimals and floating points.
Applications that intent to cater to multi-national geographies, we need to show country- specific codes and their respective currency values. That's where CurrencyPipe comes to our rescue.
The CurrencyPipe operator is used to append the country codes or currency symbol in front of the number values.
Take a look the code snippet implementing the CurrencyPipe operator:
{{ value | currency:'USD' }}
Expenses in INR:
{{ expenses | currency:'INR' }}
Let's analyze the preceding code snippet in detail:
So now that we know how to use a currency pipe operator, let's put together an example to display multiple currency and country formats.
The following is the complete component class, which implements a currency pipe operator:
import { Component } from '@angular/core';
@Component({
selector: 'currency-pipe', template: `
<h5>Built-In CurrencyPipe</h5>
<ol>
<li>
<p>Salary in USD: {{ salary | currency:'USD':true }}</p>
<p>Expenses in INR: {{ expenses | currency:'INR':false }}</p>
</li>
</ol>
`
})
export class CurrencyPipeComponent {
salary: number = 2500;
expenses: number = 1500;
}
Let's analyze the the preceding code in detail:
Launch the app, and we should see the output as shown in the following screenshot:
In this section, we learned about and implemented CurrencyPipe. In the following sections, we will keep exploring and learning about other Built-in Pipes and much more.
import { Component } from '@angular/core';
@Component({
selector: 'currency-pipe', template: `
<h5>Built-In CurrencyPipe</h5>
<ol>
<li>
<p>Salary in USD: {{ salary | currency:'USD':true }}</p>
<p>Expenses in INR: {{ expenses | currency:'INR':false }}</p>
</li>
</ol>
`
})
export class CurrencyPipeComponent {
salary: number = 2500;
expenses: number = 1500;
}
The LowercasePipe and UppercasePipe, as the name suggests, help in transforming the text into lowercase and uppercase, respectively.
Take a look at the following code snippet:
Author is Lowercase
{{authorName | lowercase }}
Author in Uppercase is
{{authorName | uppercase }}
Let's analyze the preceding code in detail:
Now that we saw how to define lowercase and uppercase pipes, it's time we create a complete component example, which implements the Pipes to show author name in both lowercase and uppercase.
Take a look at the following code snippet:
import { Component } from '@angular/core';
@Component({
selector: 'textcase-pipe', template: `
<h5>Built-In LowercasPipe and UppercasePipe</h5>
<ol>
<li>
<strong>LowercasePipe example</strong>
<p>Author in lowercase is {{authorName | lowercase}}
</li>
<li>
<strong>UpperCasePipe example</strong>
<p>Author in uppercase is {{authorName | uppercase}}
</li>
</ol>
`
})
export class TextCasePipeComponent {
authorName = "Sridhar Rao";
}
Let's analyze the preceding code in detail:
Run the application, and we should see the output as shown in the following screenshot:
In this section, you learned how to use lowercase and uppercase pipes to transform the values.
Similar to JSON filter in Angular 1.x, we have JSON Pipe, which helps us transform the string into a JSON format string.
In lowercase or uppercase pipe, we were transforming the strings; using JSON Pipe, we can transform and display the string into a JSON format string.
The general syntax is shown in the following code snippet:
<pre>{{ myObj | json }}</pre>
Now, let's use the preceding syntax and create a complete component example, which uses the JSON Pipe:
import { Component } from '@angular/core';
@Component({
template: `
<h5>Author Page</h5>
<pre>{{ authorObj | json }}</pre>
`
})
export class JSONPipeComponent {
authorObj: any; constructor() { this.authorObj = { name: 'Sridhar Rao',
website: 'http://packtpub.com', Books: 'Mastering Angular2'
};
}
}
Let's analyze the preceding code in detail:
Run the app, and we should see the output as shown in the following screenshot:
JSON is soon becoming defacto standard of web applications to integrate between services and client technologies. Hence, JSON Pipe comes in handy every time we need to transform our values to JSON structure in the view.
Slice Pipe is very similar to array slice JavaScript function. It gets a sub string from a strong certain start and end positions.
The general syntax to define a slice pipe is given as follows:
{{email_id | slice:0:4 }}
In the preceding code snippet, we are slicing the e-mail address to show only the first four characters of the variable value email_id.
Now that we know how to use a slice pipe, let's put it together in a component. The following is the complete complete code snippet implementing the slice pipe:
import { Component } from '@angular/core';
@Component({
selector: 'slice-pipe', template: `
<h5>Built-In Slice Pipe</h5>
<ol>
<li>
<strong>LowercasePipe example</strong>
<p> Email Id is {{ emailAddress }}
</li>
<li>
<strong>LowercasePipe example</strong>
<p>Sliced Email Id is {{emailAddress | slice : 0: 4}}
</li>
</ol>
`
})
export class SlicePipeComponent {
emailAddress = "test@packtpub.com";
}
Let's analyze the preceding code snippet in detail:
Run the app, and we should the output as shown in the following screenshot:
SlicePipe is certainly a very helpful Built-in Pipe specially dealing with strings or substrings.
async Pipe allows us to directly map a promises or observables into our template view. To understand async Pipe better, let me throw some light on an Observable first.
Observables are Angular-injectable services, which can be used to stream data to multiple sections in the application. In the following code snippet, we are using async Pipe as a promise to resolve the list of authors being returned:
<ul id="author-list">
<li *ngFor="let author of authors | async">
<!-- loop the object here -->
</li>
</ul>
The async pipe now subscribes to the observable (authors) and retrieve the last value. Let's look at examples of how we can use the async pipe as both promise and an observable.
Add the following lines of code in our app.component.ts file:
getAuthorDetails(): Observable<Author[]> {
return this.http.get(this.url).map((res: Response) => res.json());
}
getAuthorList(): Promise<Author[]> {
return this.http.get(this.url).toPromise().then((res: Response) =>
res.json());
}
Let's analyze the preceding code snippet in detail:
In this section, we have seen how the async pipe works. You will find it very similar to dealing with services. We can either map a promise or an observable and map the result to the template.
To summarize, we demonstrated Angular Pipes by explaining in detail about various built-in Pipes such as DatePipe, DecimalPipe, CurrencyPipe, LowercasePipe and UppercasePipe, JSON Pipe, SlicePipe, and async Pipe.
[box type="note" align="" class="" width=""]The above article is an excerpt from the book Expert Angular, written by Sridhar Rao, Rajesh Gunasundaram, and Mathieu Nayrolles. This book will help you learn everything you need to build highly scalable and robust web applications using Angular 4. What are you waiting for, check out the book now to become an expert Angular developer![/box]
Interview - Why switch to Angular for web development
Building Components Using Angular