










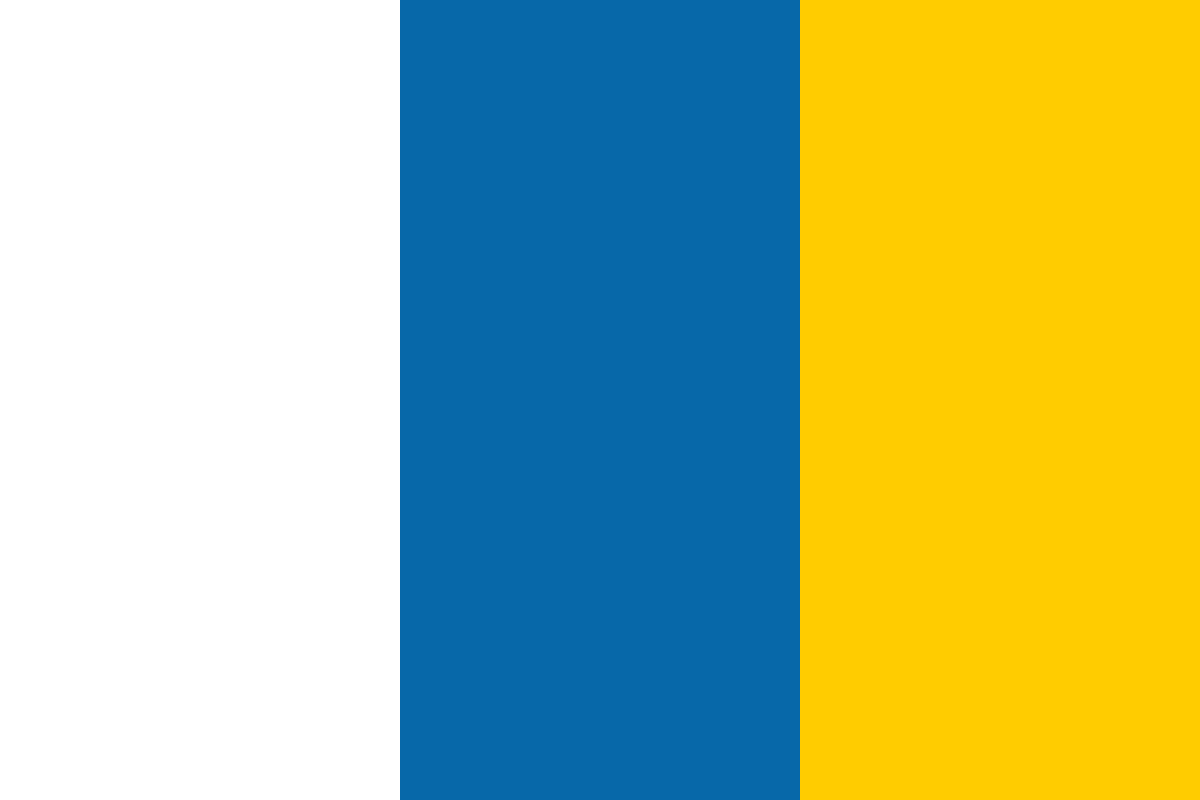

































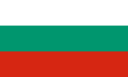








(For more resources related to this topic, see here.)
HTML5 Boilerplate provides a handy and safe way to load jQuery. With jQuery, it is vastly simple to work on writing scripts to access elements.
If you are writing custom jQuery script either to kick off a plugin you are using or to do some small interaction, put it in the main.js file in the js folder.
If you are more comfortable using other libraries, you can also load and use them in a similar way to jQuery.
The following is how we load jQuery:
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.8.2/jquery.min. js"></script> <script>window.jQuery || document.write('<script src="js/vendor/ jquery-1.8.2.min.js"></script>') </script>
Let us say, you want to use another library (like MooTools ), then look up the Google Libraries API to see if that library is available at developers.google.com/speed/libraries/. If it is available, just replace the reference with the appropriate reference from the site. For example, if we want to replace our jQuery link with a link to MooTools, we would simply replace the following code:
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min. js"> </script>
With the following line of code:
<script src="ajax.googleapis.com/ajax/libs/mootools/1.4.5/mootoolsyui- compressed.js"> </script>
We will also download Mootools' minified file to the js/vendor folder locally and replace the following code:
<script>window.jQuery||document.write('<script src="js/vendor/jquery- 1.7.2.min.js"></script>') </script>
With the following line of code:
<script>window.jQuery||document.write('<script src="js/vendor/ mootools-core-1.4.5-full-compat-yc.js"></script>') </script>
If you have not noticed it already, the website we are building is a single page site! All content that is required is found on the same page. The way our site is currently designed, it would mean clicking on one of the site navigation links would scroll roughly to the section that the navigation link refers to. We would like this interaction to be smooth. Let us use jQuery's smooth-scroll plugin to provide this.
Let us download the plugin file from the Github repository, hosted on github.com/kswedberg/jquery-smooth-scroll.
In it, we find a minimized version of the plugin (jquery.smooth-scroll.min.js) that we shall open in our text editor.
Then copy all the code and paste it within the plugins.js file.
Let us add a class name js-scrollitem to let us distinguish that this element has a script that will be used on those elements. This way, there will be a lesser chance of accidentally deleting class names that are required for interactions prompted via JavaScript.
Now, we shall write the code to invoke this plugin in the main.js file. Open the main.js file in your text editor and type:
$('.js-scrollitem').smoothScroll();
This will make all the clickable links that link to sections on the same page within the parent container with class js-scrollitem scroll smoothly with the help of the plugin. If we have used our HTML5 Boilerplate defaults correctly, adding this will be more than sufficient to get started with smooth scrolling.
Next, we would like the navigation links in the line up section to open the right-hand side line up depending on which day was clicked on. Right now, in the following screenshot, it simply shows the line up for the first day, and does not do anything else:
Let us continue editing the main.js file and add in the code that would enable this.
First, let's add the class names that we will use to control the styling, and the hiding/showing behavior within our code. The code for this functionality is as follows:
<nav class="t-tab__nav"> <a class="t-tab__navitem--active t-tab__navitemjs-tabitem" href="#day- 1">Day 1</a> <a class="t-tab__navitemjs-tabitem" href="#day-2">Day 2</a> </nav>
Now, we shall write the code that will show the element we clicked on. This code is as follows:
var $navlinks = $('#lineup .js-tabitem'); var $tabs = $('.t-tab__body'); var hiddenClass = 'hidden'; var activeClass = 't-tab__navitem--active'; $navlinks.click(function() { // our code for showing or hiding the current day's line up $(this.hash).removeClass(hiddenClass); });
By checking how we have done so far, we notice it keeps each day's line up always visible and does not hide them once done! Let us add that too, as shown in the following code snippet:
var $navlinks = $('#lineup .js-tabitem'); var $tabs = $('.t-tab__body'); var hiddenClass = 'hidden'; var activeClass = 't-tab__navitem--active'; var $lastactivetab = null; $navlinks.click(function() { var $this = $(this); //take note of what was the immediately previous tab and tab nav that was active $lastactivetab = $lastactivetab || $tabs.not('.' + hiddenClass); // our code for showing or hiding the current day's line up $lastactivetab.addClass(hiddenClass); $(this.hash).removeClass(hiddenClass); $lastactivetab = $(this.hash); return false; }
You would notice that the active tab navigation item still seems to suggest it is Day 1! Let us fix that by changing our code to do something similar with the tabbed navigation anchors, as shown in the following code snippet:
var $navlinks = $('#lineup .js-tabitem'); var $tabs = $('.t-tab__body'); var hiddenClass = 'hidden'; var activeClass = 't-tab__navitem--active'; var $lastactivetab = null; var $lastactivenav = null; $navlinks.click(function() { var $this = $(this); //take note of what was the immediately previous tab and tab nav that was active $lastactivetab = $lastactivetab || $tabs.not('.' + hiddenClass); $lastactivenav = $lastactivenav || $navlinks.filter('.' + activeClass); // our code for showing or hiding the current day's line up $lastactivetab.addClass(hiddenClass); $(this.hash).removeClass(hiddenClass); $lastactivetab = $(this.hash); // change active navigation item $lastactivenav.removeClass(activeClass); $this.addClass(activeClass); $lastactivenav = $this; return false; });
Bingo! We have our day-by-day line up ready. We now need to ensure our Google Maps iframe renders when users click on the Locate on a map link. We also want to use the same link to hide the map if the users want to do so.
First, we add some identifiable features to the anchor element used to trigger the showing/hiding of map and the iframe for the maps, as shown in the following code snippet:
<p>The festival will be held on the beautiful beaches of NgorTerrou
Bi in Dakar.
<ahref="#" class="js-map-link">Locate it on a map</a>
</p>
<iframe id="venue-map" class="hidden" width="425"
height="350" frameborder="0" scrolling="no" marginheight="0"
marginwidth="0" src="http://maps.google.com/maps?f=q&source=s
_q&hl=en&geocode=&q=ngor+terrou+bi,+dakar,+senegal&
;aq=&sll=37.0625,-95.677068&sspn=90.404249,95.976562&i
e=UTF8&hq=ngor&hnear=Terrou-Bi,+Bd+Martin+Luther+King,+Gue
ule+Tapee,+Dakar+Region,+Guediawaye,+Dakar+221,+Senegal&t=m&am
p;fll=14.751996,-17.513559&fspn=0.014276,0.011716&st=1091
46043351405611748&rq=1&ev=p&split=1&ll=14.711109,-
17.483921&spn=0.014276,0.011716&output=embed">
</iframe>
Then we use the following JavaScript to trigger the link:
$maplink = $('.js-map-link'); $maplinkText = $maplink.text(); $maplink.toggle(function() { $('#venue-map').removeClass(hiddenClass); $maplink.text('Hide Map'); }, function() { $('#venue-map').addClass(hiddenClass); $maplink.text($maplinkText); });