










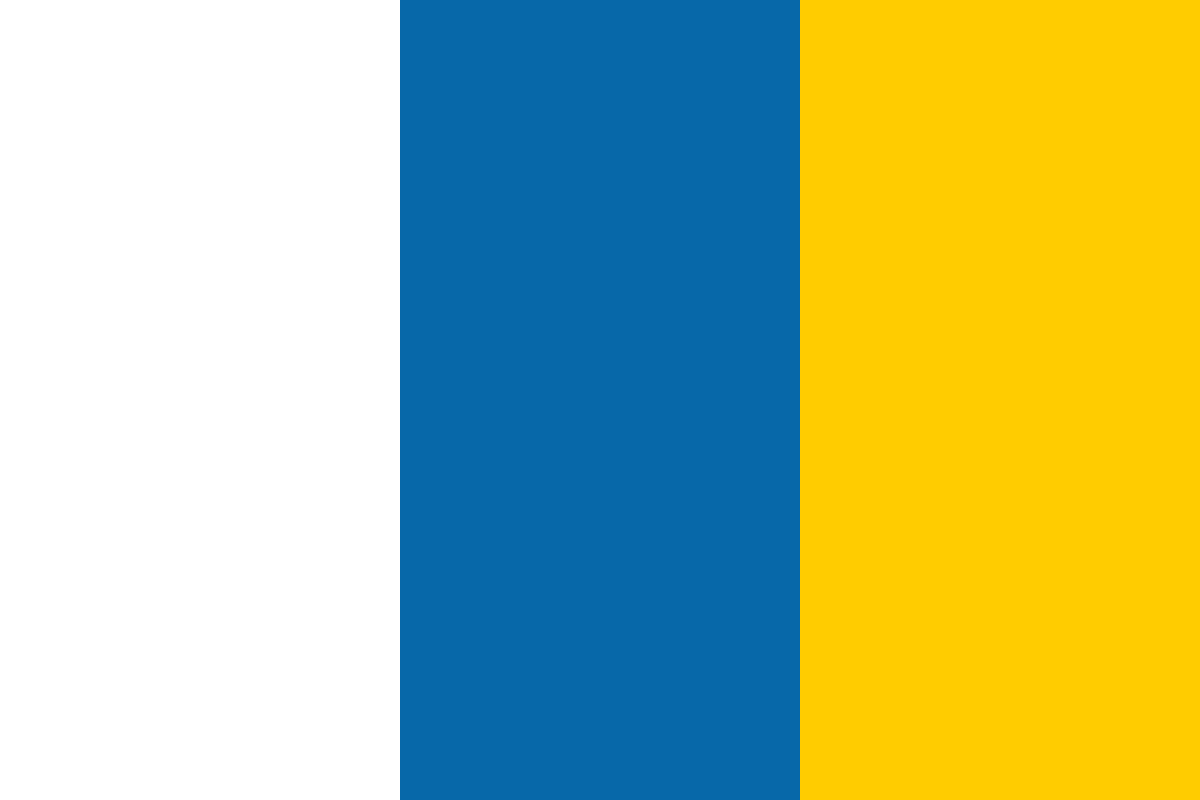

































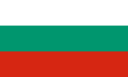








Before VectorVision was integrated, Papervision3D already had a Lines3D class for drawing 3D lines. Two differences between drawing lines with VectorShape3D and Lines3D are:
Let's take a look at how to create straight as well as curved lines with Lines3D, and how to add interactivity. The following class will serve as a template for the Lines3D examples to come:
package
{
import flash.events.Event;
import org.papervision3d.core.geom.Lines3D;
import org.papervision3d.core.geom.renderables.Line3D;
import org.papervision3d.core.geom.renderables.Vertex3D;
import org.papervision3d.materials.special.LineMaterial;
import org.papervision3d.view.BasicView;
public class Lines3DTemplate extends BasicView
{
private var lines:Lines3D;
private var easeOut:Number = 0.6;
private var reachX:Number = 0.5
private var reachY:Number = 0.5
private var reachZ:Number = 0.5;
public function Lines3DTemplate ()
{
super(stage.stageWidth,stage.stageHeight);
stage.frameRate = 40;
init();
startRendering();
}
private function init():void
{
//code to be added
}
override protected function onRenderTick(e:Event=null):void
{
var xDist:Number = mouseX - stage.stageWidth * 0.5;
var yDist:Number = mouseY - stage.stageHeight * 0.5;
camera.x += (xDist - camera.x * reachX) * easeOut;
camera.y += (yDist - camera.y * reachY) * easeOut;
camera.z += (-mouseY * 2 - camera.z ) * reachZ;
super.onRenderTick();
}
}
}
Let's first examine what happens when we draw a line using the Lines3D class.
Each line is defined by a start and an end point, both being 3D vertices. The vertices are converted into 2D space coordinates. The lineTo() method of the Flash drawing API is then used to render the line.
To create a line with the Lines3D class, we need to take these steps in the following order:
Equivalent to how Particle instances need to be added in Particles using theaddParticle() method, we add Line3D instances to a Lines3D instance in order to render them.
Lines3D has the following three methods to add Line3D instances:
Let's have a look at what they do and create some lines.
All t he following code should be added inside the init() method. First we create line material.
var blueMaterial:LineMaterial = new LineMaterial(0x0000FF);
You can pass two optional parameters as shown in the next table:
|
Parameter |
Data type |
Default value |
Description |
1 |
color |
Number |
0xFF0000 |
Defines the color of the line material using a 24 bit hexadecimal color value. |
2 |
alpha |
Number |
1 |
Sets the transparency of the material. |